Turbulent flows. Statistical description and characteristic scales#
This topic has been prepared principally based on chapter 3 of excellent Pope’s book “Turbulent Flows” ( UPC library link )
1. Random variables#
According to Wikipedia, a random variable is the outcome of a random phenomenon which, apparently, lacks a pattern or predictability.
The numpy module has a random number generator.
from numpy.random import default_rng
import numpy as np
rng = default_rng()
rng.uniform()
0.525230718052224
Every time we call this function, it will generate a different value, in this case, with a uniform distribution between 0 and 1
rng.uniform()
0.8058190818800551
We cannot predict the value, but the probability of the numbers is the same.
import matplotlib.pyplot as plt
rvar = rng.uniform(size=1000)
x_plot = np.linspace(0,1,1000)
fig,ax = plt.subplots(1,2,figsize=(16,4))
ax[0].scatter(x_plot,rvar)
ax[1].hist(rvar,bins=20,orientation="horizontal",density=True);
ax[1].set_xlim([0,5]);
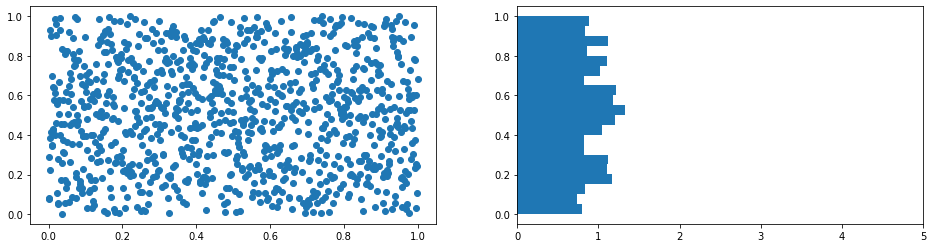
We can generate random numbers with another probability distribution, for example, a normal distribution
rnvar = rng.normal(loc=0.5,scale=0.1,size=1000)
fig,ax = plt.subplots(1,2,figsize=(16,4))
ax[0].scatter(x_plot,rnvar)
ax[1].hist(rnvar,bins=20,orientation="horizontal",density=True);
ax[1].set_xlim([0,5]);
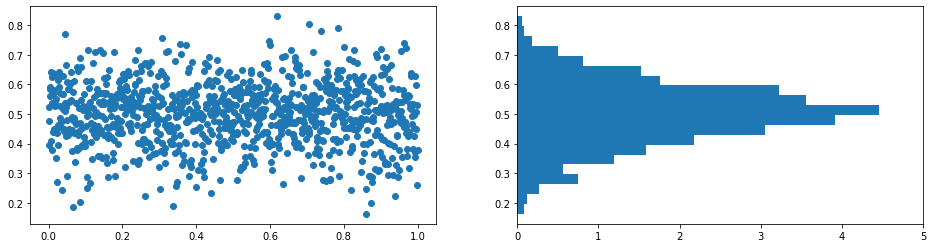
2. Lorenz equation and chaos#
One of the characteristics of turbulent flow is that it is chaotic, meaning that, after a while, it becomes random. But, how can be random a phenomenon that, like laminar flow, is described by deterministic Navier-Stokes equations? The answer lies in the initial and boundary conditions. Unlike laminar flows (like the ones we saw in microflows part), turbulent flows are very sensitive to very small changes in initial and boundary conditions: small vibrations, slight changes in temperature, pressure, surface…
Let’s see that with a typical example: Lorenz equations. Lorenz defined a system of 3 non linear ODEs:
where \(\rho\), \(\beta\) and \(\sigma\) are constant parameters. It the values \(\rho = 28.0\), \(\sigma = 10.0\) and \(\beta = 8/3\), it gives the famous Lorenz attractor.
from scipy.integrate import odeint
from mpl_toolkits.mplot3d import Axes3D
rho = 28.0
sigma = 10.0
beta = 8.0 / 3.0
def f(pos, t):
x, y, z = pos # Unpack the state vector
return sigma * (y - x), x * (rho - z) - y, x * y - beta * z # Derivatives
pos0 = [0.1, 0.1, 0.1]
t = np.arange(0.0, 100.0, 0.01)
pos = odeint(f, pos0, t)
fig = plt.figure(figsize=(10,10))
ax = plt.axes(projection="3d")
ax.plot(pos[:, 0], pos[:, 1], pos[:, 2]);
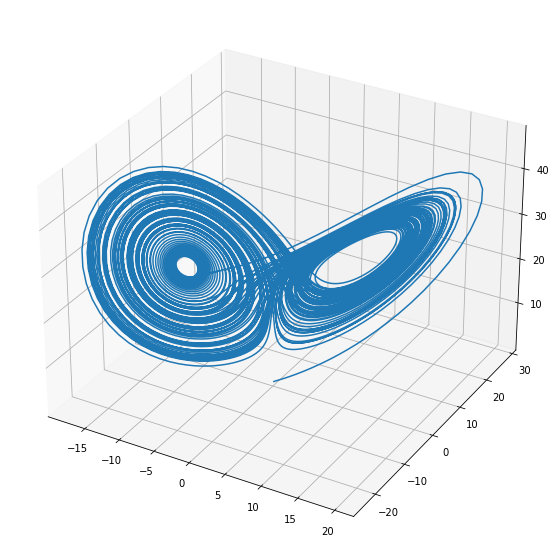
Let’s plot only the \(x\) coordinate in time
fig = plt.figure(figsize=(8,4))
ax = fig.gca()
ax.plot(t,pos[:,0])
[<matplotlib.lines.Line2D at 0x7f90440cfdc0>]
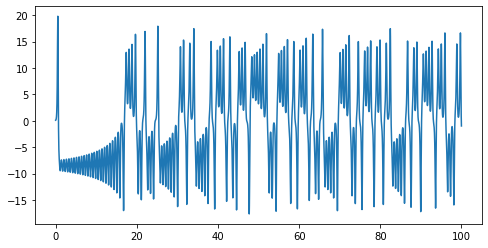
… and let’s change only slightly the initial position
pos0 = [0.1000001, 0.1, 0.1]
pos_new = odeint(f, pos0, t)
fig = plt.figure(figsize=(8,4))
ax = fig.gca()
ax.plot(t,pos_new[:,0])
[<matplotlib.lines.Line2D at 0x7f9044038d90>]
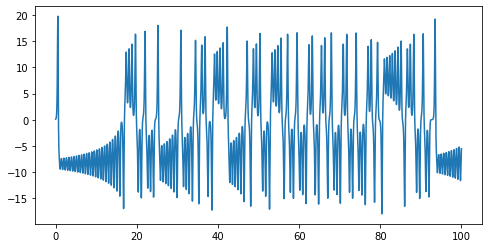
You can see that, after a while, \(x\) position changes in an unpredictable way.
We can compute this change of position in time
delta_x = pos_new[:,0] - pos[:,0]
fig = plt.figure(figsize=(8,4))
ax = fig.gca()
ax.plot(t,delta_x)
[<matplotlib.lines.Line2D at 0x7f9044022ca0>]
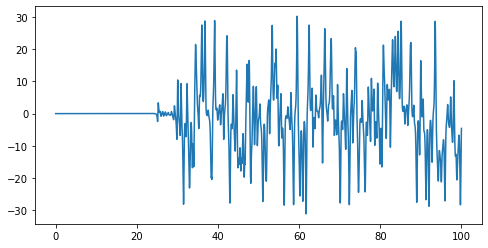
You can try playing with it, making the difference in the initial position more and more small (for instance, pos0 = [0.100000000001, 0.1, 0.1]
). When will be the two results indentical? Why?
try:
%load_ext watermark
except:
!pip install watermark
%watermark -v -m -iv
Python implementation: CPython
Python version : 3.9.12
IPython version : 8.2.0
Compiler : GCC 7.5.0
OS : Linux
Release : 5.4.0-113-generic
Machine : x86_64
Processor : x86_64
CPU cores : 8
Architecture: 64bit
matplotlib: 3.5.1
numpy : 1.21.5